How can I convert a string to a date object in JavaScript with a specific format?Gable E![gable e profile pic]()
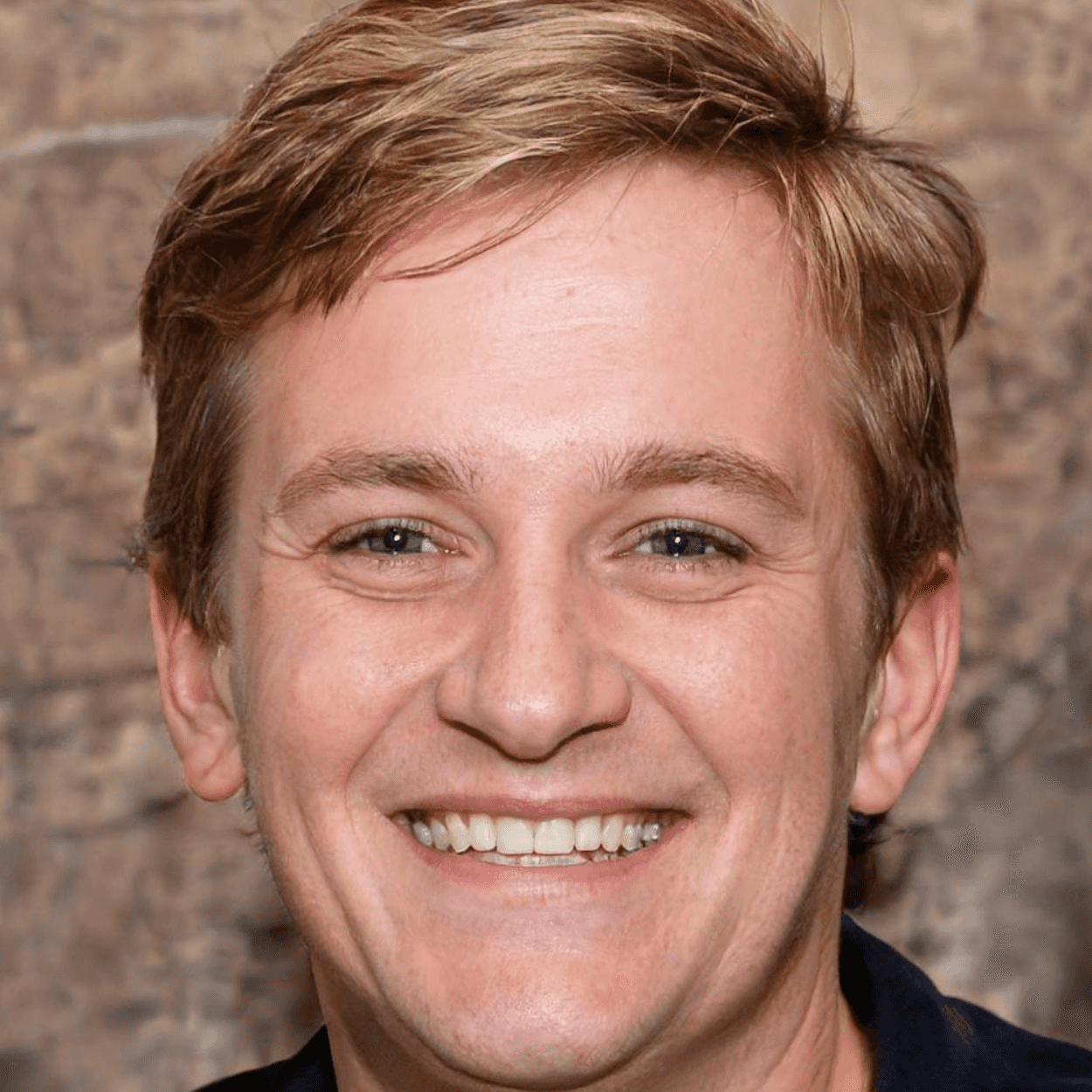
To convert a string to a date object in JavaScript with a specific format, you can use theDate
constructor and a combination of string manipulation and built-in date methods. Here's a step-by-step guide on how you can achieve this:
1. Define the input string and the desired format:
1 2 3 4
const dateString = '2023-05-18'; const format = 'YYYY-MM-DD';
2. Create a function that maps the format placeholders to their corresponding values:
1 2 3 4 5 6 7 8 9 10 11 12
function getDateValues(dateString, format) { const dateParts = dateString.split('-'); const formatParts = format.split('-'); return formatParts.reduce((values, part, index) => { const key = part.charAt(0); values[key] = parseInt(dateParts[index]); return values; }, {}); }
3. Use thegetDateValues()
function to obtain an object with the year, month, and day values:
1 2 3
const dateValues = getDateValues(dateString, format);
4. Construct the date object using the obtained values:
1 2 3
const dateObject = new Date(dateValues.YYYY, dateValues.MM - 1, dateValues.DD);
Now,dateObject
will hold the date object corresponding to the input string and format.
Note: The format placeholder letters used in thegetDateValues()
function (e.g., 'YYYY', 'MM', 'DD') can be customized to match your desired format. Make sure to adjust the function accordingly.
Here's an example of the complete code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
function getDateValues(dateString, format) { const dateParts = dateString.split('-'); const formatParts = format.split('-'); return formatParts.reduce((values, part, index) => { const key = part.charAt(0); values[key] = parseInt(dateParts[index]); return values; }, {}); } const dateString = '2023-05-18'; const format = 'YYYY-MM-DD'; const dateValues = getDateValues(dateString, format); const dateObject = new Date(dateValues.YYYY, dateValues.MM - 1, dateValues.DD); console.log(dateObject);
In the example above, the input string is'2023-05-18'
, and the format is'YYYY-MM-DD'
. The resulting date object corresponds to May 18, 2023. Adjust the code according to your specific format requirements.
Similar Questions
How can I convert a string to a date object in a specific format in JavaScript?
How can I convert a string to a date object in a specific timezone in JavaScript?
How can I convert a string to a date object in JavaScript?
How can I convert a string to a datetime object in a specific format in Python?
How can I convert a string to a datetime object in a specific format in Python?
How can I convert a string to an object in JavaScript?
How can I convert a string to a number with a specific base in JavaScript?
How can I convert a string to a nested object in JavaScript?
How can I convert an object to a specific class in JavaScript?
How can I convert an object to a string in JavaScript?
How can I convert a JSON string to an object in JavaScript?
How can I convert a JSON string to an object in JavaScript?
How can I convert a string to title case in JavaScript?
How can I convert a string to title case in JavaScript?
How can I convert a JavaScript object to a specific class instance?
How can I convert a JavaScript object to a specific class instance?
How can I convert a number to a string with a specific number of decimal places in JavaScript?
How can I convert a JavaScript string to a Blob object?
How can I convert an object to an XML string in JavaScript?
How can I convert an object to a JSON string in JavaScript with indentation?